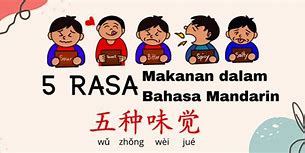
Channel-Specific Response Variations#
To specify different response variations depending on which channel the user is connected to, use channel-specific response variations.
In the following example, the channel key makes the first response variation channel-specific for the slack channel while the second variation is not channel-specific:
- text: "Which game would you like to play on Slack?"
- text: "Which game would you like to play?"
Make sure the value of the channel key matches the value returned by the name() method of your input channel. If you are using a built-in channel, this value will also match the channel name used in your credentials.yml file.
When your assistant looks for suitable response variations under a given response name, it will first try to choose from channel-specific variations for the current channel. If there are no such variations, the assistant will choose from any response variations which are not channel-specific.
In the above example, the second response variation has no channel specified and can be used by your assistant for all channels other than slack.
For each response, try to have at least one response variation without the channel key. This allows your assistant to properly respond in all environments, such as in new channels, in the shell and in interactive learning.
Validating Form Input#
After extracting a slot value from user input, you can validate the extracted slots. By default Rasa Open Source only validates if any slot was filled after requesting a slot.
Forms no longer raise ActionExecutionRejection if nothing is extracted from the user’s utterance for any of the required slots.
You can implement a Custom Action validate_
- validate_restaurant_form
When the form is executed it will run your custom action.
This custom action can extend FormValidationAction class to simplify
the process of validating extracted slots. In this case, you need to write functions
named validate_
The following example shows the implementation of a custom action which validates that the slot named cuisine is valid.
from typing import Text, List, Any, Dict
from rasa_sdk import Tracker, FormValidationAction
from rasa_sdk.executor import CollectingDispatcher
from rasa_sdk.types import DomainDict
class ValidateRestaurantForm(FormValidationAction):
def name(self) -> Text:
return "validate_restaurant_form"
def cuisine_db() -> List[Text]:
"""Database of supported cuisines"""
return ["caribbean", "chinese", "french"]
def validate_cuisine(
dispatcher: CollectingDispatcher,
) -> Dict[Text, Any]:
"""Validate cuisine value."""
if slot_value.lower() in self.cuisine_db():
return {"cuisine": slot_value}
return {"cuisine": None}
You can also extend the Action class and retrieve extracted slots with tracker.slots_to_validate to fully customize the validation process.
Session configuration#
A conversation session represents the dialogue between the assistant and the user. Conversation sessions can begin in three ways:
the user begins the conversation with the assistant,
the user sends their first message after a configurable period of inactivity, or
a manual session start is triggered with the /session_start intent message.
You can define the period of inactivity after which a new conversation session is triggered in the domain under the session_config key.
Available parameters are:
The default session configuration looks as follows:
session_expiration_time: 60 # value in minutes, 0 means infinitely long
carry_over_slots_to_new_session: true # set to false to forget slots between sessions
This means that if a user sends their first message after 60 minutes of inactivity, a new conversation session is triggered, and that any existing slots are carried over into the new session. Setting the value of session_expiration_time to 0 means that sessions will not end (note that the action_session_start action will still be triggered at the very beginning of conversations).
A session start triggers the default action action_session_start. Its default implementation moves all existing slots into the new session. Note that all conversations begin with an action_session_start. Overriding this action could for instance be used to initialize the tracker with slots from an external API call, or to start the conversation with a bot message. The docs on Customizing the session start action shows you how to do that.
Session configuration#
A conversation session represents the dialogue between the assistant and the user. Conversation sessions can begin in three ways:
the user begins the conversation with the assistant,
the user sends their first message after a configurable period of inactivity, or
a manual session start is triggered with the /session_start intent message.
You can define the period of inactivity after which a new conversation session is triggered in the domain under the session_config key.
Available parameters are:
The default session configuration looks as follows:
session_expiration_time: 60 # value in minutes, 0 means infinitely long
carry_over_slots_to_new_session: true # set to false to forget slots between sessions
This means that if a user sends their first message after 60 minutes of inactivity, a new conversation session is triggered, and that any existing slots are carried over into the new session. Setting the value of session_expiration_time to 0 means that sessions will not end (note that the action_session_start action will still be triggered at the very beginning of conversations).
A session start triggers the default action action_session_start. Its default implementation moves all existing slots into the new session. Note that all conversations begin with an action_session_start. Overriding this action could for instance be used to initialize the tracker with slots from an external API call, or to start the conversation with a bot message. The docs on Customizing the session start action shows you how to do that.
The config key in the domain file maintains the store_entities_as_slots parameter. This parameter is used only in the context of reading stories and turning them into trackers. If the parameter is set to True, this will result in slots being implicitly set from entities if applicable entities are present in the story. When an entity matches the from_entity slot mapping, store_entities_as_slots defines whether the entity value should be placed in that slot. Therefore, this parameter skips adding an explicit slot_was_set step manually in the story. By default, this behaviour is switched on.
You can turn off this functionality by setting the store_entities_as_slots parameter to false:
store_entities_as_slots: false
If you're looking for information on the config.yml file, check out the docs on Model Configuration.
A story is a representation of a conversation between a user and an AI assistant, converted into a specific format where user inputs are expressed as intents (and entities when necessary), while the assistant's responses and actions are expressed as action names.
Here's an example of a dialogue in the Rasa story format:
- story: collect restaurant booking info # name of the story - just for debugging
- intent: greet # user message with no entities
- action: utter_ask_howcanhelp
- intent: inform # user message with entities
- action: utter_on_it # action that the bot should execute
- action: utter_ask_cuisine
- action: utter_ask_num_people
While writing stories, you do not have to deal with the specific contents of the messages that the users send. Instead, you can take advantage of the output from the NLU pipeline, which lets you use just the combination of an intent and entities to refer to all the possible messages the users can send to mean the same thing.
It is important to include the entities here as well because the policies learn to predict the next action based on a combination of both the intent and entities (you can, however, change this behavior using the use_entities attribute).
All actions executed by the bot, including responses are listed in stories under the action key.
You can use a response from your domain as an action by listing it as one in a story. Similarly, you can indicate that a story should call a custom action by including the name of the custom action from the actions list in your domain.
During training, Rasa does not call the action server. This means that your assistant's dialogue management model doesn't know which events a custom action will return.
Because of this, events such as setting a slot or activating/deactivating a form have to be explicitly written out as part of the stories. For more info, see the documentation on Events.
Slot events are written under slot_was_set in a story. If this slot is set inside a custom action, add the slot_was_set event immediately following the custom action call. If your custom action resets a slot value to None, the corresponding event for that would look like this:
- story: set slot to none
# ... other story steps
- action: my_custom_action
There are three kinds of events that need to be kept in mind while dealing with forms in stories.
A form action event (e.g. - action: restaurant_form) is used in the beginning when first starting a form, and also while resuming the form action when the form is already active.
A form activation event (e.g. - active_loop: restaurant_form) is used right after the first form action event.
A form deactivation event (e.g. - active_loop: null), which is used to deactivate the form.
In order to get around the pitfall of forgetting to add events, the recommended way to write these stories is to use interactive learning.
Custom Slot Mappings#
If none of the predefined Slot Mappings fit your use
case, you can use the
Custom Action validate_
If you're using the Rasa SDK we recommend you to extend the provided FormValidationAction. When using the FormValidationAction, three steps are required to extract customs slots:
The following example shows the implementation of a form which extracts a slot outdoor_seating in a custom way, in addition to the slots which use predefined mappings. The method extract_outdoor_seating sets the slot outdoor_seating based on whether the keyword outdoor was present in the last user message.
from typing import Dict, Text, List, Optional, Any
from rasa_sdk import Tracker
from rasa_sdk.executor import CollectingDispatcher
from rasa_sdk.forms import FormValidationAction
class ValidateRestaurantForm(FormValidationAction):
def name(self) -> Text:
return "validate_restaurant_form"
async def required_slots(
slots_mapped_in_domain: List[Text],
dispatcher: "CollectingDispatcher",
domain: "DomainDict",
) -> Optional[List[Text]]:
required_slots = slots_mapped_in_domain + ["outdoor_seating"]
return required_slots
async def extract_outdoor_seating(
self, dispatcher: CollectingDispatcher, tracker: Tracker, domain: Dict
) -> Dict[Text, Any]:
text_of_last_user_message = tracker.latest_message.get("text")
sit_outside = "outdoor" in text_of_last_user_message
return {"outdoor_seating": sit_outside}
By default the FormValidationAction will automatically set the requested_slot to the first slot specified in required_slots which is not filled.
Test Conversation Format#
The test conversation format is a format that combines both NLU data and stories into a single file for evaluation. Read more about this format in Testing Your Assistant.
This format is only used for testing and cannot be used for training.
End-to-end training is an experimental feature. We introduce experimental features to get feedback from our community, so we encourage you to try it out! However, the functionality might be changed or removed in the future. If you have feedback (positive or negative) please share it with us on the Rasa Forum.
With end-to-end training, you do not have to deal with the specific intents of the messages that are extracted by the NLU pipeline or with separate utter_ responses in the domain file. Instead, you can include the text of the user messages and/or bot responses directly in your stories. See the training data format for detailed description of how to write end-to-end stories.
You can mix training data in the end-to-end format with labeled training data which has intents and actions specified: Stories can have some steps defined by intents/actions and other steps defined directly by user or bot utterances.
We call it end-to-end training because policies can consume and predict actual text. For end-to-end user inputs, intents classified by the NLU pipeline and extracted entities are ignored.
Only Rule Policy and TED Policy allow end-to-end training.
RulePolicy uses simple string matching during prediction. Namely, rules based on user text will only match if the user text strings inside your rules and input during prediction are identical.
TEDPolicy passes user text through an additional Neural Network to create hidden representations of the text. In order to obtain robust performance you need to provide enough training stories to capture a variety of user texts for any end-to-end dialogue turn.
Rasa policies are trained for next utterance selection. The only difference to creating utter_ response is how TEDPolicy featurizes bot utterances. In case of an utter_ action, TEDPolicy sees only the name of the action, while if you provide actual utterance using bot key, TEDPolicy will featurize it as textual input depending on the NLU configuration. This can help in case of similar utterances in slightly different situations. However, this can also make things harder to learn because the fact that different utterances have similar texts make it easier for TEDPolicy to confuse these utterances.
End-to-end training requires significantly more parameters in TEDPolicy. Therefore, training an end-to-end model might require significant computational resources depending on how many end-to-end turns you have in your stories.
Dari Wikipedia bahasa Indonesia, ensiklopedia bebas
Unjuk rasa atau demonstrasi (disingkat menjadi "demo") adalah sebuah gerakan protes yang dilakukan sekumpulan orang di hadapan umum. Unjuk rasa biasanya dilakukan untuk menyatakan pendapat kelompok tersebut atau penentang kebijakan yang dilaksanakan suatu pihak atau dapat pula dilakukan sebagai sebuah upaya penekanan secara politik oleh kepentingan kelompok.
Unjuk rasa umumnya dilakukan oleh kelompok mahasiswa dan orang-orang yang tidak setuju dengan pemeritah dan yang menentang kebijakan pemerintah. Namun unjuk rasa juga dilakukan oleh kelompok-kelompok lainnya dengan tujuan lainnya.
Di Indonesia, unjuk rasa menjadi hal yang umum sejak jatuhnya rezim kekuasaan Soeharto pada tahun 1998, dan unjuk rasa menjadi simbol kebebasan berekspresi di negara ini. Unjuk rasa terjadi hampir setiap hari di berbagai bagian di Indonesia, khususnya Jakarta.
adjar.id - Dalam bahasa Inggris, terdapat berbagai istilah yang bisa digunakan untuk mendeskripsikan rasa makanan atau food flavour.
Contohnya untuk mendeskripsikan rasa asam, kita bisa menggunakan kata acidic untuk rasa yang sangat asam.
Sedangkan rasa asam yang wajar, kita bisa menggunakan istilah sour.
Nah, rasa asam yang cenderung sedikit atau tipis, kita bisa menggunakan istilah tart.
Apa lagi istilah yang bisa digunakan untuk mendeskripsikan rasa makanan dalam bahasa Inggris, ya?
Istilah Bahasa Inggris untuk Mendeskripsikan Rasa Makanan
Pahit = Rasa tajam yang kuat yang tidak manis
Baca Juga: 20 Tekstur Makanan atau Food Texture dalam Bahasa Inggris
Pahit manis = Mencicipi pahit dan manis pada saat bersamaan
Pedas = Mengandung banyak bumbu yang menimbulkan rasa panas di mulut
Kuat = Dibiarkan mengembangkan rasa kuat yang menyenangkan
Ringan = Tidak memiliki rasa yang kuat
Matang = Memiliki rasa yang kuat
Baca Juga: Contoh Percakapan Mendeskripsikan Makanan dalam Bahasa Inggris
Kuat dan kaya rasa = Memiliki banyak rasa
Gurih = Terasa garam atau bumbu dan tidak manis
Dibumbui dengan baik = Mengandung bumbu untuk meningkatkan cita rasa
Tajam = Memiliki rasa yang kuat dan pahit
Asam = Rasa asam seperti lemon
Pedas = Memiliki rasa pedas yang kuat
Baca Juga: Percakapan Memesan Makanan dengan Menggunakan Bahasa Inggris
Manis dan asam = Mengandung rasa manis dan asam
Seperti sirup = Kental, manis, dan lengket
Asam = Rasa yang sedikit asam
Tidak asin = Tidak dibumbui dengan garam
Berair = Pucat atau tidak kuat rasa
Itu dia beberapa istilah yang bisa digunakan untuk mendeskripsikan rasa makanan, Adjarian.
Baca Juga: Cara Memuji Rasa Makanan dalam Bahasa Inggris serta Contoh Percakapannya
In Rasa, your domain defines the universe in which your assistant operates. Specifically, it lists:
If you are building an NLU-based assistant, refer to the Domain documentation to see how intents, entities, slot mappings, and slot featurization can be configured in your domain.
Using a Custom Action to Ask For the Next Slot#
As soon as the form determines which slot has to be filled next by the user, it will
execute the action utter_ask_
from typing import Dict, Text, List
from rasa_sdk import Tracker
from rasa_sdk.events import EventType
from rasa_sdk.executor import CollectingDispatcher
from rasa_sdk import Action
class AskForSlotAction(Action):
def name(self) -> Text:
return "action_ask_cuisine"
self, dispatcher: CollectingDispatcher, tracker: Tracker, domain: Dict
) -> List[EventType]:
dispatcher.utter_message(text="What cuisine?")
Here is a full example of a domain, taken from the concertbot example:
influence_conversation: false
influence_conversation: false
influence_conversation: true
- text: "Sorry, I didn't get that, can you rephrase?"
- text: "You're very welcome."
- text: "I am a bot, powered by Rasa."
- text: "I can help you find concerts and venues. Do you like music?"
- text: "Awesome! You can ask me things like \"Find me some concerts\" or \"What's a good venue\""
- action_search_concerts
- action_search_venues
- action_show_concert_reviews
- action_show_venue_reviews
- action_set_music_preference
session_expiration_time: 60 # value in minutes
carry_over_slots_to_new_session: true
Persistence of Slots during Coexistence#
In Coexistence of NLU-based and CALM systems the action action_reset_routing resets all slots and hides events from featurization for the NLU-based system policies to prevent them from seeing events that originated while CALM was active. However, you might want to share some slots that both CALM and the NLU-based system should be able to use. One use case for these slots are basic user profile slots. Both the NLU-based system and CALM should likely be able to know whether a user is logged in or not, what their user name is, or what channel they are using. If you are storing this kind of data in slots you can annotate those slot definitions with the option shared_for_coexistence: True.
shared_for_coexistence: True
shared_for_coexistence: True
In the coexistence mode, if the option shared_for_coexistence is NOT set to true, it invalidates the reset_after_flow_ends: False property in the flow definition. In order for the slot value to be retained throughout the conversation, the shared_for_coexistence must be set to true.
Checkpoints and OR statements#
Checkpoints and OR statements should be used with caution, if at all. There is usually a better way to achieve what you want by using Rules or the ResponseSelector.
You can use checkpoints to modularize and simplify your training data. Checkpoints can be useful, but do not overuse them. Using lots of checkpoints can quickly make your example stories hard to understand, and will slow down training.
Here is an example of stories that contain checkpoints:
- story: beginning of flow
- action: action_ask_user_question
- checkpoint: check_asked_question
- story: handle user affirm
- checkpoint: check_asked_question
- action: action_handle_affirmation
- checkpoint: check_flow_finished
- story: handle user deny
- checkpoint: check_asked_question
- action: action_handle_denial
- checkpoint: check_flow_finished
- checkpoint: check_flow_finished
- action: utter_goodbye
Unlike regular stories, checkpoints are not restricted to starting with user input. As long as the checkpoint is inserted at the right points in the main stories, the first event can be a custom action or a response as well.
Another way to write shorter stories, or to handle multiple intents or slot events the same way, is to use an or statement. For example, if you ask the user to confirm something, and you want to treat the affirm and thankyou intents in the same way. The story below will be converted into two stories at training time:
- action: utter_ask_confirm
- action: action_handle_affirmation
You can also use or statements with slot events. The following means the story requires that the current value for the name slot is set and is either joe or bob:
- action: utter_greet
or statements can be useful, but if you are using a lot of them, it is probably better to restructure your domain and/or intents. Overusing OR statements will slow down training.